|
| 1 | +--- |
| 2 | +sidebar_position: 1 |
| 3 | +sidebar_label: 'Case Study: Refactor to rxEffects' |
| 4 | +title: 'Case Study: Refactor to rxEffects' |
| 5 | +--- |
| 6 | + |
| 7 | +## Problem |
| 8 | + |
| 9 | +Let's get the problem it solves into code so we can refactor it. |
| 10 | + |
| 11 | +We start with the side effect and 2 ways to execute it: |
| 12 | + |
| 13 | +```typescript |
| 14 | +@Component({ |
| 15 | + // ... |
| 16 | +}) |
| 17 | +export class MyComponent { |
| 18 | + // The side effect (`console.log`) |
| 19 | + private effectFn = (num: number) => console.log('number: ' + num); |
| 20 | + // The interval triggers our function including the side effect |
| 21 | + private trigger$ = interval(1000); |
| 22 | + |
| 23 | + constructor() { |
| 24 | + // [#1st approach] The subscribe's next callback it used to wrap and execute the side effect |
| 25 | + this.trigger$.subscribe(this.effectFn); |
| 26 | + |
| 27 | + // [#2nd approach] `tap` is used to wrap and execute the side effect |
| 28 | + this.trigger$.pipe(tap(this.effectFn)).subscribe(); |
| 29 | + } |
| 30 | +} |
| 31 | +``` |
| 32 | + |
| 33 | +As we introduced a memory leak we have to setup some boilerplate code to handle the cleanup logic: |
| 34 | + |
| 35 | +```ts |
| 36 | +import { takeUntilDestroyed } from '@angular/core/rxjs-interop'; |
| 37 | + |
| 38 | +@Component({ |
| 39 | + // ... |
| 40 | +}) |
| 41 | +export class MyComponent { |
| 42 | + // The side effect (`console.log`) |
| 43 | + private effectFn = (num: number) => console.log('number: ' + num); |
| 44 | + // The interval triggers our function including the side effect |
| 45 | + private trigger$ = interval(1000); |
| 46 | + |
| 47 | + constructor() { |
| 48 | + this.trigger$.effect$ |
| 49 | + .pipe( |
| 50 | + takeUntilDestroyed() |
| 51 | + // ⚠ Notice: Don't put any operator after takeUntil to avoid potential subscription leaks |
| 52 | + ) |
| 53 | + .subscribe(this.effectFn); |
| 54 | + } |
| 55 | +} |
| 56 | +``` |
| 57 | + |
| 58 | +There are already a couple of things that are crucial: |
| 59 | + |
| 60 | +- unsubscribe on destroy |
| 61 | +- having the `takeUntil` operator as last operator in the chain |
| 62 | + |
| 63 | +Another way would be using the `subscription` to run the cleanup logic. |
| 64 | + |
| 65 | +```ts |
| 66 | +@Component({ |
| 67 | + // ... |
| 68 | +}) |
| 69 | +export class MyComponent implements OnDestroy { |
| 70 | + // The side effect (`console.log`) |
| 71 | + private effectFn = (num: number) => console.log('number: ' + num); |
| 72 | + // The interval triggers our function including the side effect |
| 73 | + private trigger$ = interval(1000); |
| 74 | + // ⚠ Notice: The created subscription must be stored to `unsubscribe` later |
| 75 | + private readonly subscription: Subscription; |
| 76 | + |
| 77 | + constructor() { |
| 78 | + // ⚠ Notice: Never forget to store the subscription |
| 79 | + this.subscription = this.trigger$.subscribe(this.effectFn); |
| 80 | + } |
| 81 | + |
| 82 | + ngOnDestroy(): void { |
| 83 | + // ⚠ Notice: Never forget to cleanup the subscription |
| 84 | + this.subscription.unsubscribe(); |
| 85 | + } |
| 86 | +} |
| 87 | +``` |
| 88 | + |
| 89 | +## Solution |
| 90 | + |
| 91 | +In RxAngular we think the essential problem here is the call to `subscribe` itself. All `Subscription`s need to get unsubscribed manually which most of the time produces heavy boilerplate or even memory leaks if ignored or did wrong. |
| 92 | +Like `rxState`, `rxEffects` is a local instance created by a component and thus tied to the components life cycle. |
| 93 | +We can manage `Observables` as reactive triggers for side effects or manage `Subscription`s which internally hold side effects. |
| 94 | +To also provide an imperative way for developers to unsubscribe from the side effect `register` returns an "asyncId" similar to `setTimeout`. |
| 95 | +This can be used later on to call `unregister` and pass the async id retrieved from a previous `register` call. This stops and cleans up the side effect when invoked. |
| 96 | + |
| 97 | +As an automatism any registered side effect will get cleaned up when the related component is destroyed. |
| 98 | + |
| 99 | +Using `rxEffects` to maintain side-effects |
| 100 | + |
| 101 | +```ts |
| 102 | +import { rxEffects } from '@rx-angular/state/effects'; |
| 103 | + |
| 104 | +@Component({}) |
| 105 | +export class MyComponent { |
| 106 | + // The side effect (`console.log`) |
| 107 | + private effectFn = (num: number) => console.log('number: ' + num); |
| 108 | + // The interval triggers our function including the side effect |
| 109 | + private trigger$ = interval(1000); |
| 110 | + |
| 111 | + private effects = rxEffects(({ register }) => { |
| 112 | + register(this.trigger$, this.effectFn); |
| 113 | + }); |
| 114 | +} |
| 115 | +``` |
| 116 | + |
| 117 | +> **⚠ Notice:** |
| 118 | +> Avoid calling `register`, `unregister` , `subscribe` inside the side-effect function. (here named `doSideEffect`) |
| 119 | +
|
| 120 | +## Impact |
| 121 | + |
| 122 | +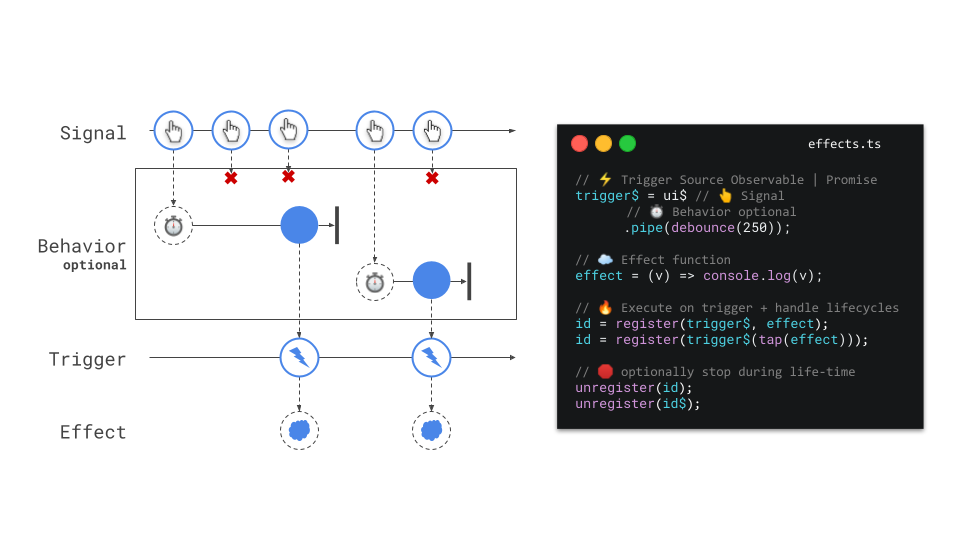 |
| 123 | + |
| 124 | +Compared to common approaches `rxEffects` does not rely on additional decorators or operators. |
| 125 | +In fact, it removes the necessity of the `subscribe`. |
| 126 | + |
| 127 | +This results in less boilerplate and a good guidance to resilient and ergonomic component architecture. |
| 128 | +Furthermore, the optional imperative methods help to glue third party libs and a mixed but clean code style in Angular. |
0 commit comments