|
1 |
| -[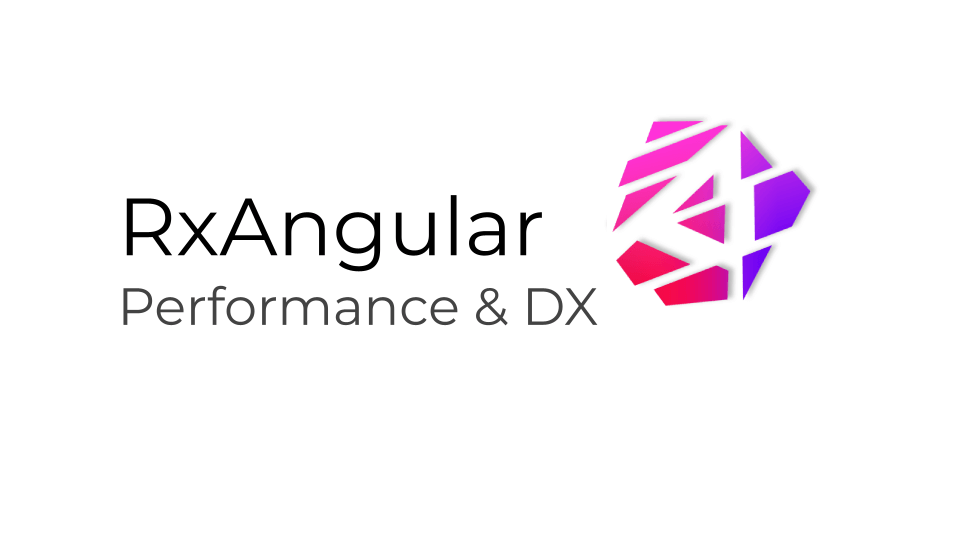](https://rx-angular.io/) |
| 1 | +[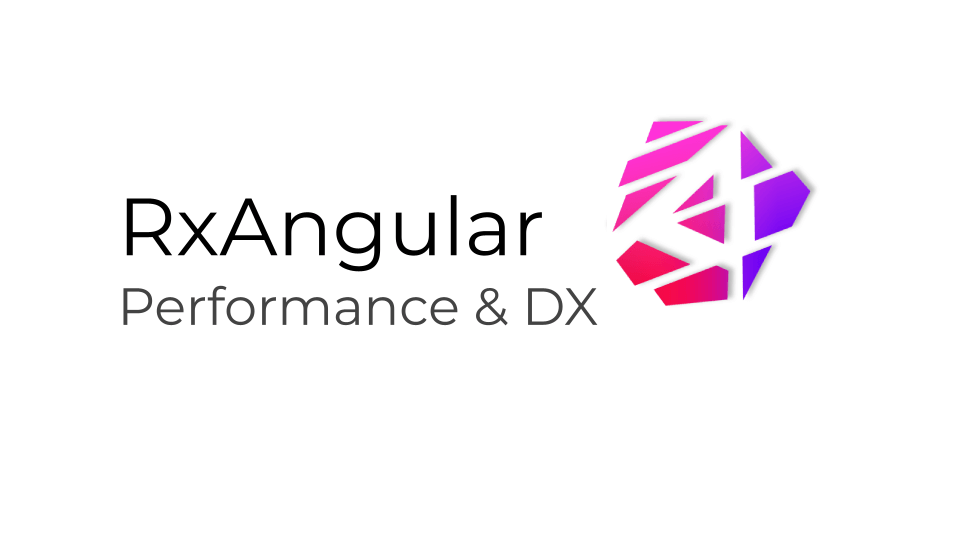](https://rx-angular.io/) |
2 | 2 |
|
3 |
| -# RxAngular  |
| 3 | +# RxAngular  |
4 | 4 |
|
5 |
| -RxAngular offers a comprehensive toolset for handling fully reactive Angular applications with the main focus on runtime |
6 |
| -performance and template rendering. |
| 5 | +> RxAngular offers a comprehensive toolkit for handling fully reactive Angular applications with the main focus on runtime performance, template rendering, and Developer eXperience. |
7 | 6 |
|
8 |
| -RxAngular is divided into different packages: |
| 7 | +## Packages |
| 8 | + |
| 9 | +RxAngular is made up of different packages that work together or standalone. |
| 10 | + |
| 11 | +| Package | Description | Version | |
| 12 | +| --------------------------------------------------------------------- | -------------------------------------------------------------------------------------------------------------------- | ------------------------------------------------------------------------------------------------------------------------------------- | |
| 13 | +| [@rx-angular/state](https://rx-angular.io/docs/state) | A powerful state management library, providing a fully reactive way to manage state in components and services. | [](https://www.npmjs.com/package/%40rx-angular%2Fstate) | |
| 14 | +| [@rx-angular/template](https://rx-angular.io/docs/template) | A set of directives and pipes designed for high-performance and non-blocking rendering for large-scale applications. | [](https://www.npmjs.com/package/%40rx-angular%2Ftemplate) | |
| 15 | +| [@rx-angular/cdk](https://rx-angular.io/docs/cdk) | A Component Development Kit for high-performance and ergonomic Angular UI libs and large-scale applications. | [](https://www.npmjs.com/package/%40rx-angular%2Fcdk) | |
| 16 | +| [@rx-angular/eslint-plugin](https://rx-angular.io/docs/eslint-plugin) | A set of ESLint rules for building reactive, performant, and zone-less Angular applications. | [](https://www.npmjs.com/package/%40rx-angular%2Feslint-plugin) | |
| 17 | + |
| 18 | +This repository holds a set of helpers that are aiming to provide: |
| 19 | + |
| 20 | +- fully reactive applications |
| 21 | +- fully or partially zone-less applications |
| 22 | +- high-performance and non-blocking rendering |
| 23 | + |
| 24 | +## Getting Started |
| 25 | + |
| 26 | +### Using `@rx-angular/template` |
9 | 27 |
|
10 |
| -- [📦@rx-angular/state](https://rx-angular.io/docs/state) |
11 |
| -- [📦@rx-angular/template](https://rx-angular.io/docs/template) |
12 |
| -- [📦@rx-angular/cdk](https://rx-angular.io/docs/cdk) |
13 |
| -- [📦@rx-angular/eslint-plugin](https://rx-angular.io/docs/eslint-plugin) |
| 28 | +This is an example of how to use the `*rxLet` directive to bind an Observable value to the template. In this example, the component defines a property `time$`, which is an Observable that emits a value every second using the `timer` operator. The emitted values are mapped to the current time string using the `map` operator which is then displayed in the template using `*rxLet`. |
14 | 29 |
|
15 |
| -Used together, you get a powerful tool for developing high-performance angular applications with or without NgZone. |
| 30 | +```ts |
| 31 | +@Component({ |
| 32 | + selector: 'app-time', |
| 33 | + standalone: true, |
| 34 | + imports: [LetDirective], |
| 35 | + template: ` |
| 36 | + <ng-container *rxLet="time$; let value"> |
| 37 | + {{ value }} |
| 38 | + </ng-container> |
| 39 | + `, |
| 40 | +}) |
| 41 | +export class TimeComponent { |
| 42 | + time$ = timer(0, 1000).pipe(map(() => new Date().toTimeString())); |
| 43 | +} |
| 44 | +``` |
16 | 45 |
|
17 |
| -This repository holds a set of helpers to create **fully reactive** as well as **fully zone-less** applications. |
| 46 | +To learn more about @rx-angular/template and its capabilities, check out the official documentation at [https://rx-angular.io/docs/template](https://rx-angular.io/docs/template). |
18 | 47 |
|
19 |
| -## Benefits |
| 48 | +### Using `@rx-angular/state` |
20 | 49 |
|
21 |
| -- 🔥 It's fast & performance focused: exceptional runtime speed & small bundle size |
22 |
| -- ✔ Easy upgrade paths: migration scripts included since beta! `ng update @rx-angular/{cdk | template | state}` |
23 |
| -- ✔ Lean and simple: No boilerplate guaranteed |
24 |
| -- ✔ Well typed and tested |
25 |
| -- ✔ Backwards compatible: support for Angular > v11 |
| 50 | +In this example, we're creating a fully reactive counter component. We define the state using an interface and use the `RxState` service to manage it. We also define two actions to increment and decrement the count and use the `connect` method to update the state in response to these actions. Finally, we use the `select` method to display the count property of the state in the template. |
| 51 | + |
| 52 | +```ts |
| 53 | +interface CounterState { |
| 54 | + count: number; |
| 55 | +} |
| 56 | + |
| 57 | +interface CounterActions { |
| 58 | + increment: void; |
| 59 | + decrement: void; |
| 60 | +} |
| 61 | + |
| 62 | +@Component({ |
| 63 | + selector: 'app-counter', |
| 64 | + standalone: true, |
| 65 | + imports: [PushPipe], |
| 66 | + template: ` |
| 67 | + <p>Count: {{ count$ | push }}</p> |
| 68 | + <button (click)="actions.increment()">Increment</button> |
| 69 | + <button (click)="actions.decrement()">Decrement</button> |
| 70 | + `, |
| 71 | + providers: [RxState, RxActionFactory], |
| 72 | +}) |
| 73 | +export class CounterComponent { |
| 74 | + readonly count$ = this.state.select('count'); |
| 75 | + readonly actions = this.actionFactory.create(); |
| 76 | + |
| 77 | + constructor( |
| 78 | + private readonly state: RxState<CounterState>, |
| 79 | + private readonly actionFactory: RxActionFactory<CounterActions> |
| 80 | + ) { |
| 81 | + this.state.set({ count: 0 }); |
| 82 | + this.state.connect(this.actions.increment$, (state) => ({ |
| 83 | + count: state.count + 1, |
| 84 | + })); |
| 85 | + this.state.connect(this.actions.decrement$, (state) => ({ |
| 86 | + count: state.count - 1, |
| 87 | + })); |
| 88 | + } |
| 89 | +} |
| 90 | +``` |
| 91 | + |
| 92 | +To learn more about @rx-angular/state and its capabilities, check out the official documentation at [https://rx-angular.io/docs/state](https://rx-angular.io/docs/state). |
26 | 93 |
|
27 | 94 | ## Used by
|
28 | 95 |
|
@@ -57,12 +124,12 @@ This repository holds a set of helpers to create **fully reactive** as well as *
|
57 | 124 |
|
58 | 125 | - [📚 Official docs](https://www.rx-angular.io/)
|
59 | 126 | - [ Discord channel](https://discord.com/invite/XWWGZsQ)
|
| 127 | +- [ Slack](https://join.slack.com/t/rxangular/shared_invite/zt-1tn1hivnp-FemQzop69HI7~wlPSqDjKQ) |
60 | 128 |
|
61 |
| -## Packages |
| 129 | +## Contributing |
| 130 | + |
| 131 | +We welcome contributions from the community to help improve RxAngular! To get started, please take a look at our contribution guidelines in the [CONTRIBUTING.md](CONTRIBUTING.md) file. We appreciate your help in making RxAngular better for everyone. |
62 | 132 |
|
63 |
| -Find details in the links to the official docs below for installation and setup instructions, examples and resources. |
| 133 | +## License |
64 | 134 |
|
65 |
| -- [📦@rx-angular/state](https://rx-angular.io/docs/state) - Imperative & Reactive Component State-Management |
66 |
| -- [📦@rx-angular/template](https://rx-angular.io/docs/template) - High-Performance Non-Blocking Rendering |
67 |
| -- [📦@rx-angular/cdk](https://rx-angular.io/docs/cdk) - Component Development Kit |
68 |
| -- [📦@rx-angular/eslint-plugin](https://rx-angular.io/docs/eslint-plugin) - ESLint Plugin |
| 135 | +This project is MIT licensed. |
0 commit comments