-
-
Notifications
You must be signed in to change notification settings - Fork 9.6k
[OptionsResolver] Add a new method addNormalizer and normalization hierarchy #30371
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Merged
Conversation
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
9621750
to
d280f5b
Compare
Better diff for reviewers https://github.com/symfony/symfony/pull/30371/files?utf8=%E2%9C%93&diff=unified&w=1 |
It seems that our friend @carsonbot was not working 3 days ago :) Status: Needs review |
src/Symfony/Component/OptionsResolver/Debug/OptionsResolverIntrospector.php
Outdated
Show resolved
Hide resolved
@nicolas-grekas comments addressed, thanks! |
nicolas-grekas
approved these changes
Mar 7, 2019
HeahDude
approved these changes
Mar 7, 2019
Adding doc PR reference symfony/symfony-docs#11103 |
BTW missing |
fabpot
approved these changes
Mar 31, 2019
Thank you @yceruto. |
fabpot
added a commit
that referenced
this pull request
Mar 31, 2019
…ormalization hierarchy (yceruto) This PR was squashed before being merged into the 4.3-dev branch (closes #30371). Discussion ---------- [OptionsResolver] Add a new method addNormalizer and normalization hierarchy | Q | A | ------------- | --- | Branch? | master | Bug fix? | no | New feature? | yes | BC breaks? | no | Deprecations? | no | Tests pass? | yes | Fixed tickets | #30310 | License | MIT | Doc PR | symfony/symfony-docs#11103 ### 3rd-party package <details><summary>Given: (CLICK ME)</summary> <p> Generic type: ```php class FooType extends AbstractType { private $registry; public function __construct(ManagerRegistry $registry) { $this->registry = $registry; } // buildForm ... public function configureOptions(OptionsResolver $resolver): void { $resolver->setRequired('class'); $resolver->setDefaults([ 'em' => null, 'query' => null, ]); $resolver->setAllowedTypes('em', ['null', 'string']); $resolver->setAllowedTypes('query', ['null', 'callable']); $resolver->setNormalizer('em', function (Options $options, $em) { if (null !== $em) { return $this->registry->getManager($em); } return $this->registry->getManagerForClass($options['class']); }); $resolver->setNormalizer('query', function (Options $options, $query) { if (\is_callable($query)) { $query = $query($options['em']->getRepository($options['class'])); if (!$query instanceof Query) { throw new UnexpectedTypeException($query, 'Doctrine\ORM\Query'); } } return $query; }); } } ``` </p> </details> ### App context <details><summary>Before (CLICK ME)</summary> <p> Normalizing the new allowed value will require to override the parent's normalizer: ```php class BarType extends AbstractType { private $registry; public function __construct(ManagerRegistry $registry) { $this->registry = $registry; } // buildForm ... public function configureOptions(OptionsResolver $resolver): void { $resolver->addAllowedTypes('em', 'Doctrine\ORM\EntityManagerInterface'); $resolver->setNormalizer('em', function (Options $options, $em) { if ($em instanceof EntityManagerInterface) { return $em; } if (null !== $em) { return $this->registry->getManager($em); } return $this->registry->getManagerForClass($options['class']); }); $resolver->addAllowedTypes('query', 'string'); $resolver->setNormalizer('query', function (Options $options, $query) { if (\is_callable($query)) { $query = $query($options['em']->getRepository($options['class'])); if (!$query instanceof Query) { throw new UnexpectedTypeException($query, 'Doctrine\ORM\Query'); } } if (\is_string($query)) { $query = $options['em']->createQuery($query); } return $query; }); } public function getParent() { return FooType::class; } } ``` </p> </details> <details><summary>After (CLICK ME)</summary> <p> The new normalizer is added to the stack and it'll receive the previously normalized value or if `forcePrepend = true` the validated value: ```php class BarType extends AbstractType { // buildForm ... public function configureOptions(OptionsResolver $resolver): void { $resolver->addAllowedTypes('em', 'Doctrine\ORM\EntityManagerInterface'); $resolver->addNormalizer('em', function (Options $options, $em) { if ($em instanceof EntityManagerInterface) { return $em; } return $em; }, true); // $forcePrepend = true (3rd argument) $resolver->addAllowedTypes('query', 'string'); $resolver->addNormalizer('query', function (Options $options, $query) { if (\is_string($query)) { $query = $options['em']->createQuery($query); } return $query; }); } public function getParent() { return FooType::class; } } ``` </p> </details> Commits ------- cf41254 [OptionsResolver] Add a new method addNormalizer and normalization hierarchy
javiereguiluz
added a commit
to symfony/symfony-docs
that referenced
this pull request
Apr 5, 2019
…od (yceruto) This PR was merged into the master branch. Discussion ---------- [OptionsResolver] Documenting the new addNormalizer method symfony/symfony#30371 Commits ------- a2e6c2a Documenting the new method addNormalizer in OptionsResolver component
fabpot
added a commit
that referenced
this pull request
Apr 15, 2019
…nd (yceruto) This PR was merged into the 4.3-dev branch. Discussion ---------- [Form] Show all option normalizers on debug:form command | Q | A | ------------- | --- | Branch? | master | Bug fix? | no | New feature? | yes | BC breaks? | no | Deprecations? | no | Tests pass? | yes | Fixed tickets | - | License | MIT Follow-up #30371 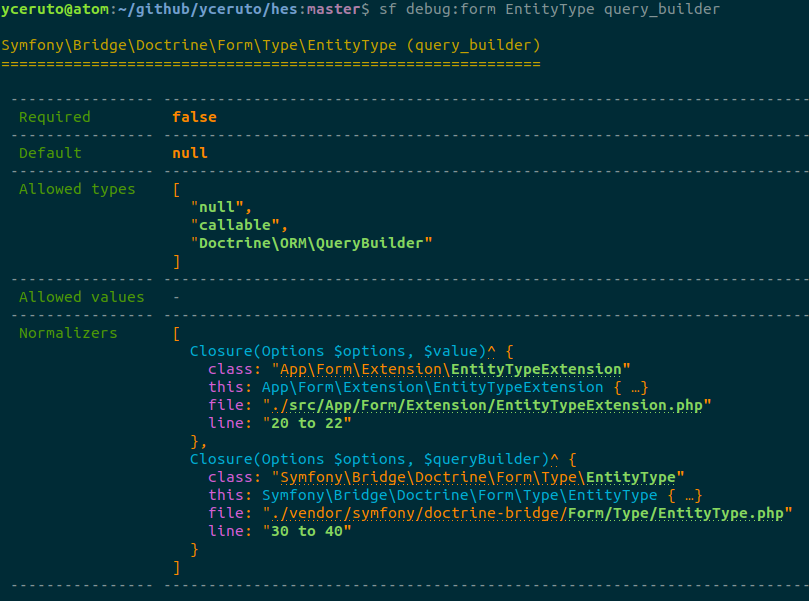 Commits ------- f1d3bc0 Show all option normalizers on debug:form command
Merged
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Add this suggestion to a batch that can be applied as a single commit.
This suggestion is invalid because no changes were made to the code.
Suggestions cannot be applied while the pull request is closed.
Suggestions cannot be applied while viewing a subset of changes.
Only one suggestion per line can be applied in a batch.
Add this suggestion to a batch that can be applied as a single commit.
Applying suggestions on deleted lines is not supported.
You must change the existing code in this line in order to create a valid suggestion.
Outdated suggestions cannot be applied.
This suggestion has been applied or marked resolved.
Suggestions cannot be applied from pending reviews.
Suggestions cannot be applied on multi-line comments.
Suggestions cannot be applied while the pull request is queued to merge.
Suggestion cannot be applied right now. Please check back later.
3rd-party package
Given: (CLICK ME)
Generic type:
App context
Before (CLICK ME)
Normalizing the new allowed value will require to override the parent's normalizer:
After (CLICK ME)
The new normalizer is added to the stack and it'll receive the previously normalized value or if
forcePrepend = true
the validated value: